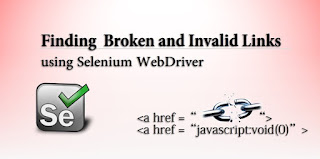
Let’s see some of the HTTP status codes.
- 200 – Valid Link
- 404 – Link not found
- 400 – Bad request
- 401 – Unauthorized
- 500 – Internal Error
Here is the sample program to find broken links
package com.selcukes.scripts; import java.net.HttpURLConnection; import java.net.URL; import java.util.List; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; public class BrokenLinks { public static WebDriver driver; public static void main(String args[]) { System.setProperty("webdriver.gecko.driver", "src/main/resources/geckodriver.exe"); driver = new FirefoxDriver(); driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); driver.get("http://techyworks.blogspot.in/"); String strPageTitle = driver.getTitle(); System.out.println("Page Title:" + strPageTitle); links.stream().filter(link -> link.getAttribute("href") != null).forEach((link) ->; { isLinkBroken(link.getAttribute("href")); }); } private static void isLinkBroken(String urlLink) { String response; try { URL link = new URL(urlLink); HttpURLConnection httpConn = (HttpURLConnection) link.openConnection(); httpConn.setConnectTimeout(2000); httpConn.connect(); response = httpConn.getResponseMessage(); httpConn.disconnect(); System.out.println("URL: " + urlLink + " - " + response); } catch (Exception e) { System.out.println("URL: " + urlLink + " - Invalid"); e.printStackTrace(); } } }
0 Comments